The approach to close a position in MQL4 and MQL5 is similar. However, the syntax might slightly differ. MQL4 uses the function OrderClose() while MQL5 uses the function PositionClose() from the library CTrade. CTrade is a class that belongs to a standard library provided by MetaTrader 5. CTrade contains trade functions that help developers easily gain access to trade manipulation, such as modifying a trade, deleting an order, or closing a position. CTrade requires to be declared to use its functions.
MQL5
Declare CTrade to get access to the trade functions.
Line 10: At the top of your MQL code, declare the CTrade library. #include <Trade\Trade.mqh>
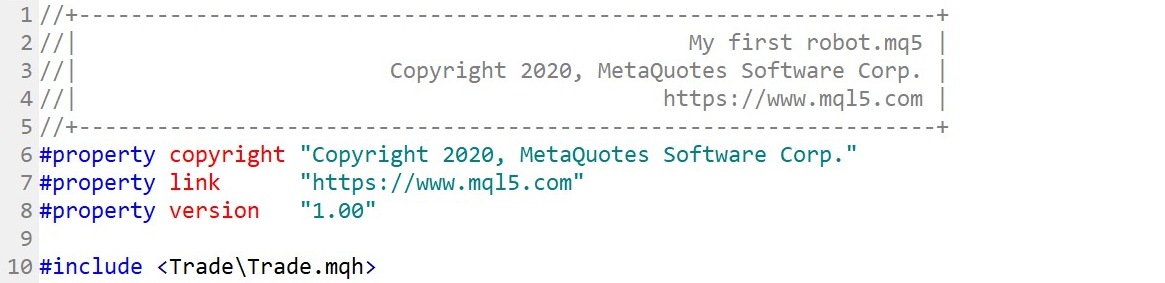
Note
- To close an active trade, use the function OrderClose() and PositionClose() in MQL4 and MQL5 respectively.
- To remove a pending order, which can be a buy stop, buy limit, sell stop and sell limit, use the function OrderDelete().
MQL5 has clearly defined the difference between an order and a position, unlike MQL4, and this is reflected in the way classes and libraries are organized in MQL5.
- Orders are pending orders that have not yet been filled.
- Positions are active trades on the market.
MQL5 CTrade (Most important functions) | |
Functions to manage orders | |
OrderOpen() | Open a pending order with specific parameters. |
OrderModify() | Modify existing pending orders. |
OrderDelete() | Remove a pending order. |
Functions to manage positions | |
PositionOpen() | Open a position with specific parameters. |
PositionModify() | Modify a position specified by symbol or position ticket ID. |
PositionClose() | Close a position specified by symbol or position ticket ID. |
MQL4 OrderClose() parameters
This function is used to close market orders. For pending orders, use the function OrderDelete(). |
|
int ticket | ticket |
double lots | volume, lot size |
double price | close Price |
int slippage | slippage |
color arrow_color | color |
true | false | OrderClose() returns true if the order has successfully been closed. |
MQL4 OrderDelete() parameters
This function is used to close or delete pending orders. |
|
int ticket | ticket |
color arrow_color | color |
true | false | OrderDelete() returns true if the pending order has successfully been deleted. |
How to close a pending order?
MQL4
To close or delete a pending order, use the OrderDelete() function

MQL5
To close or delete a pending order, use the OrderDelete() function. In order to use the OrderDelete() function, you have to declare the CTrade library.
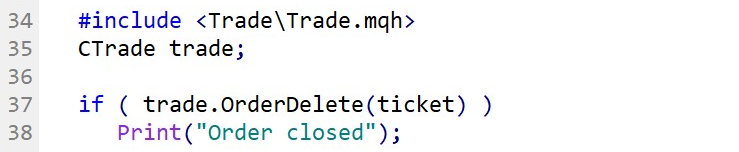
Fig.104 Close a market order with MQL5.
How to close a market order or an active trade?
MQL4
To close an order, use the OrderClose() function. Provide the order ticket ID, volume, and price. The last parameter is optional.

MQL5
To close an order, simply call the PositionClose() function. Provide the order ticket ID. Note that you may declare the CTrade library to access trade functions.
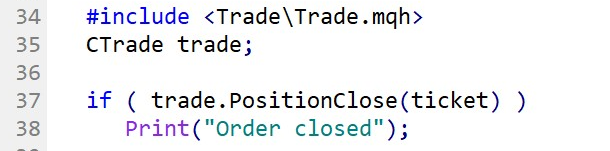
Coding tips:
Use the order ticket id instead of the symbol to define the order or trade you want to close. Delete or close an order with ticker id is a safer approach because ticket id is a unique identifier and therefore may not affect by mistake another order with the same symbol.