MQL4 and MQL5 provide a group of functions named “trade functions” that allow developers to access and manipulate everything related to trades and orders. Before we deep dive into each of the available functions, we will highlight some of the key notions you need to know, particularly if you are writing your code with MQL5. MQL5 defines trades in three distinct terms: order, deal, and position.
Order, deal, position, and history
Order deal, position, and history are the terms that define the type of transactions that are strictly applied in MQL5. MQL4, on the other hand, does not require the definition of the type of trade as all the transactions are defined as “Order.”
Order: An order is a directive given to the broker to buy or sell a financial instrument. There are two main types of orders: Market and Pending. Market orders are orders initiated at the current market price, while pending orders are executed at the specified price level. In MQL5, to interact with orders, use trade functions that start with the keyword “Order,” for instance Order{xxx}().
Deal: A deal is the commercial exchange of a financial security. A deal can be opened as a market order execution or pending order initialization. An order can have multiple deals; for instance, if a trader wishes to execute an order of 10 lots of EUR/USD, the order can be filled with 10 deals of 1 lot of EUR/USD. The deal term applies only to MQL5. Note that deals can be only accessed as transaction history. To interact with deals’ history transactions, use trade functions that start with the keyword “HistoryDeal,” for instance, HistoryDeal{xxx}().
Position: A position is a trade obligation. A position is the current running trade that has been executed. The term “position” applies only to MQL5. To interact with positions, use trade functions that start with the keyword “Position,” for instance, Position{xxx}().
History: Another notion that MQL5 brings is the transaction type “History.” History is basically closed transactions. The history term applies exclusively to MQL5, and to access the history of transactions, use trade functions that start with the keyword “History,” for instance, History{xxx}().
Note
- MQL4 and MQL5 trade functions work in the same methodology. When called, the function returns the value of the requested value.
- There is only one differentiation between MQL4 and MQL5. MQL4 requires the selection of a position before a trade function can be successfully exploited. To select a position or a pending order in MQL4, call the function OrderSelect() prior to calling any trade functions.
- MQL4 does not make any distinction between pending orders and market orders, unlike MQL5, which defines 3 types of entries: orders, positions, and deals. Hence, MQL5 functions may use these exact terms for their functions.
Trade functions
You can find the complete list of trade functions in the MQL4 and MQL5 codebase. In the list below, we have included the most important trade functions that we believe are essential and cover the creation of advanced trading robots.
MQL4 | MQL5 |
OrderClose()
closes opened order. OrderCloseBy() closes an opened order by another opposite opened order. OrderClosePrice() returns close price of the currently selected order. OrderCloseTime() returns close time of the currently selected order. OrderComment() returns comment of the currently selected order. OrderCommission() returns calculated commission of the currently selected order. OrderDelete() deletes the previously opened pending order. OrderLots() returns the number of lots of the selected order. OrderMagicNumber() returns an identifying (magic) number of the currently selected order. OrderModify() modifies the characteristics of the previously opened or pending orders. OrderOpenPrice() returns the open price of the currently selected order. OrderOpenTime() returns the open time of the currently selected order. OrderPrint() prints information about the selected order in the log. OrderProfit() returns the profit of the currently selected order. OrderSelect() is the function that selects an order for further processing. OrderSend() is the main function used to open an order or place a pending order. OrdersHistoryTotal() returns the number of closed orders in the account history loaded into the terminal. OrderStopLoss() returns stop loss value of the currently selected order. OrdersTotal() returns the number of market and pending orders. OrderSwap() returns swap value of the currently selected order. OrderSymbol() returns symbol name of the currently selected order. OrderTakeProfit() returns take the profit value of the currently selected order. OrderTicket() returns the ticket number of the currently selected order. OrderType() returns order operation type of the currently selected order. |
OrdersTotal()
returns the number of orders. OrderGetTicket() return the ticket of a corresponding order. OrderSelect() selects an order for the further working with it. OrderGetDouble() returns the requested property of the order (double). OrderGetInteger returns the requested property of the order (datetime or int). OrderGetString returns the requested property of the order (string). |
PositionsTotal()
returns the number of open positions. PositionGetSymbol() returns the symbol corresponding to the open position. PositionSelect() chooses an open position for further working with it. PositionSelectByTicket() selects a position to work with by the ticket number specified in it. PositionGetDouble() returns the requested property of an open position (double). PositionGetInteger() returns the requested property of an open position (datetime or int). PositionGetString() returns the requested property of an open position (string). PositionGetTicket() returns the ticket of the position with the specified index in the list of open positions. |
|
HistorySelect()retrieves the history of transactions and orders for the specified period of the server time.
HistorySelectByPosition() requests the history of deals with a specified position identifier. HistoryOrderSelect() selects an order in the history for the further working with it. HistoryOrdersTotal() returns the number of orders in the history. HistoryOrderGetTicket() returns the number ticket of a corresponding order in the history. HistoryOrderGetDouble() returns the requested property of an order in the history (double). HistoryOrderGetInteger() returns the requested property of an order in the history (datetime or int). HistoryOrderGetString() returns the requested property of an order in the history (string). HistoryDealSelect() selects a deal in the history for further calling it through appropriate functions. HistoryDealsTotal() returns the number of deals in the history. HistoryDealGetTicket() returns the ticket of a corresponding deal in the history. HistoryDealGetDouble() returns the requested property of a deal in the history (double). HistoryDealGetInteger() returns the requested property of a deal in the history (datetime or int). HistoryDealGetString() returns the requested property of a deal in the history (string). |
Case study: Orders, positions data manipulation
Particularities for MQL4 OrderSelect() function:
bool OrderSelect(
int index, // index or order ticket
int select, // flag
int pool=MODE_TRADES // mode
);
To distinguish between the opened trades, pending trades and transactions history, provide the parameter SELECT_BY_POS and MODE_TRADES (default value can be omitted). Instead, use MODE_HISTORY to query transaction history (closed and canceled order).
Get information from pending orders
MQL5
The overall concept of this algorithm is applicable for deals and positions and works as follow:
- Obtain the total number of orders with the function OrdersTotal().
- Iterate through all the orders, by their indexes from the list of orders or positions.
- Store into the cache various orders data using the OrderGetTicket(), OrderGetDouble(), OrderGetInteger(), and OrderGetString() functions.
- Store the data in variables for reusability and data manipulation.
MQL4
Example of collecting order ticket ID, open price, open date, and symbol from a pending order. Note that the process to collect information from an active position or trade is the same.
Get information from an active trade/position
MQL5
This is the standard concept to access opened trades/positions.
- Obtain the total number of positions, using the PositionsTotal() function.
- Iteration through all the positions by their indexes from the list.
- Collect the data with trade functions and store the values in variables.
MQL4
Example of collecting order ticket id, open price, open date, and symbol from an opened trade.
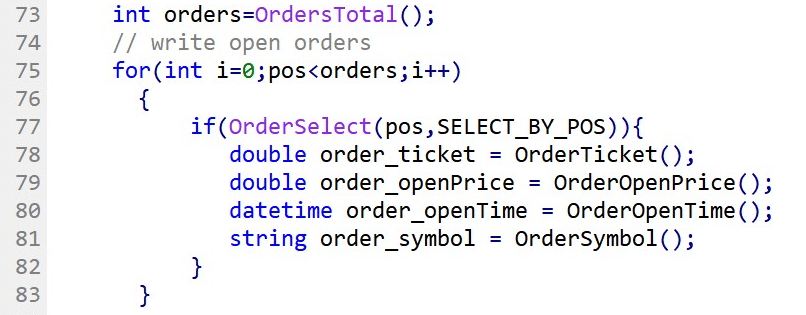
Get information from historical transactions
MQL5
This is the standard concept to access to transactions history:
- Obtain the total number of history orders, using the HistoryOrdersTotal() function.
- Iteration through all the closed trades by their indexes from the list.
- Collect the data with trade functions and store the values in variables.
- Use trade functions that start with HistoryOrders{xxx}() to retrieve information related to closed orders and trades. Use HistoryDeals{xxx}() to get information related to closed deals.
MQL4
The concept of transaction history in MQL4 is like the methodology to collect pending orders and opened trades. There are a few differences which are:
- Line 71: To get the total number of orders in the transaction history, use the function OrdersHistoryTotal() instead of OrdersTotal().
- Line 74: Specify the scope with the parameter MODE_HISTORY.
- Collect the data from the trade properties with the trade functions, OrderOpenPrice(), OrderOpenTime(), OrderSymbol() etc.
Coding tips:
When you call trade functions, you may assign the returned value to a variable to allow reusability of the data and provide a more readable code. Also, this practice is more efficient as you call the function only once to get the data.