Understanding the concept of event handling functions and ticks is crucial if you develop trading algorithms, particularly with MQL4 and MQL5. You may already get to know about ticks and tick charts, but you may not plainly grasp the significance these ticks have in the development of expert advisors. Before we dive into how to structure your code to accommodate decision-rule-based trading with indicators, we will explain the basic framework of event-handling functions and ticks. Once you clearly understand these theories, everything will be straightforward.
What is a tick, and why is it an important concept for trading robots?
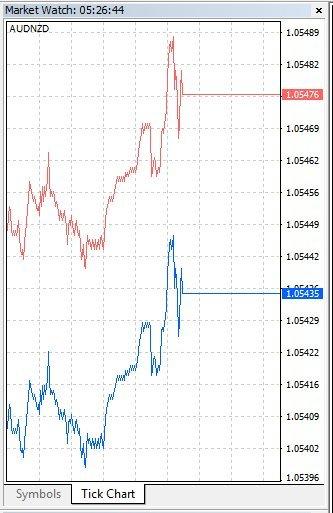
A tick can be simply defined as a change of the price of a financial instrument or commonly named symbol. The way tick works in MetaTrader is a price signal is sent from the MetaTrader server to all the connected client terminals as a feed.
The notion of a tick can be appreciated better with the help of the tick chart. A tick chart is a line chart that shows the evolution of the price through time, and you can see the tick chart for a symbol from the MetaTrader’s Market Watch panel (Fig.114).
To access the tick chart, right-click on the selected symbol. A tick chart is the fastest time frame, and it represents the real-time price change of an asset.
It is essential to know that the notion of a tick is not connected to a normal time interval. Varying on the date, time, and market conditions, you can find numerous ticks happening in one second or not even a tick in an hour. During a calm market, ticks are not frequent and, conversely, during volatile market hours, you may see many ticks being received in a short duration.
The concept of ticks is very important for the Expert Advisor. Because the Expert Advisor will execute code instruction at every tick with the main event-handling function OnTick(). Hence, the indicator’s values and conditions are evaluated at each generated tick; therefore, the logic of the algorithm will be mainly operated from that particular function.
MQL4 and MQL5 main event handling functions
The foundation of the MQL trading robot, commonly called Expert Advisor, is the Event Handling Function. Expert Advisors run perpetually unless you or the program decides to interrupt the operations. When the Expert Advisor is operating, it has some predefined functions that check the various events. In MetaTrader 4, MQL4 language, there are eight standard event-handling functions. In MetaTrader 5, MQL5 language, on the other hand, provides 14 standard event-handling functions.
The objective of this guide is to help you be productive quickly, therefore, we will only cover the three most important event handling functions that are more than enough to build a sophisticated trading robot.
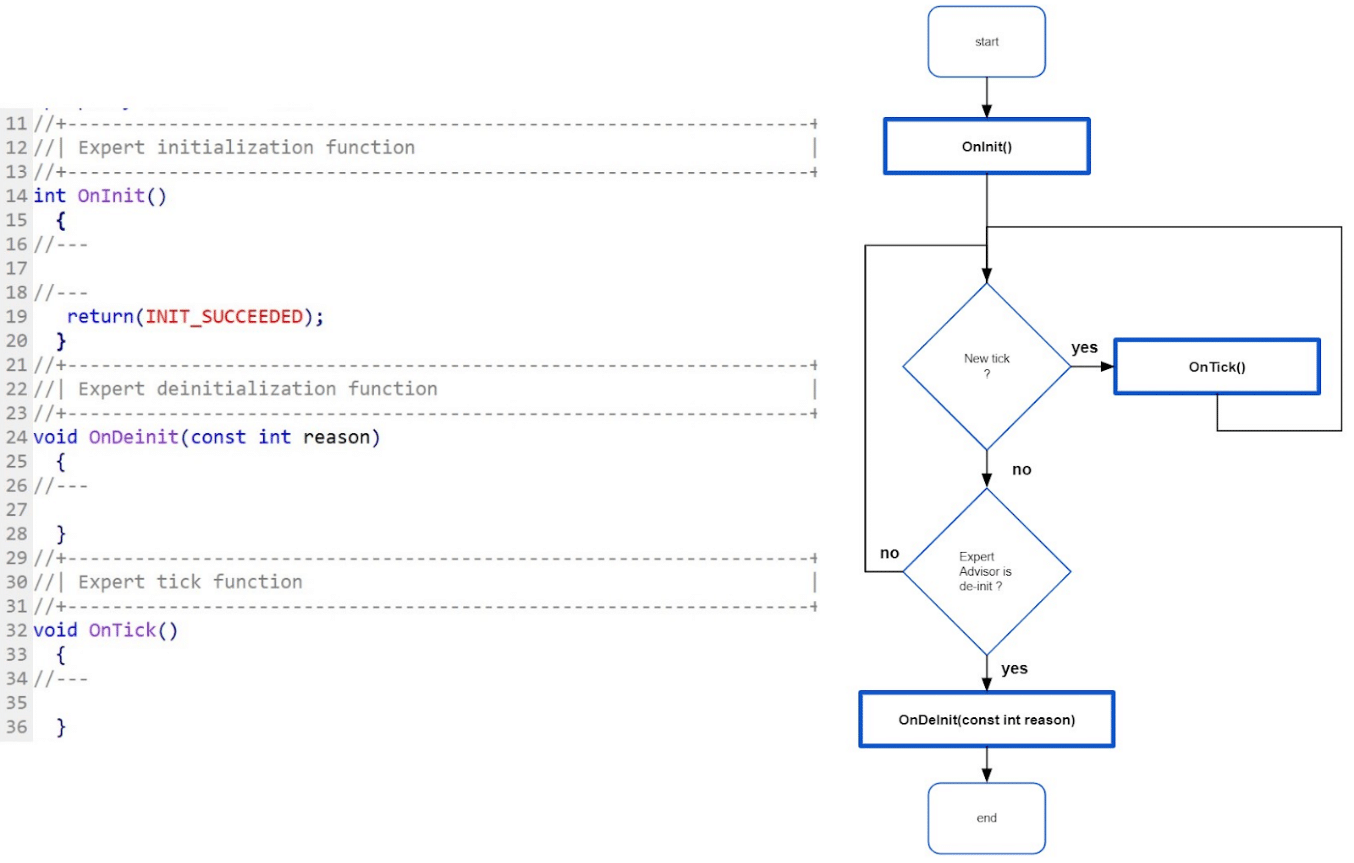
When you build a new Expert Advisor and keep all the default settings, so the MetaEditor will generate the three main event-handling functions, OnInit, OnDeInit, and OnTick. If you examine fig.115, the logic of an Expert Advisor is clear – when you start it, it will run the OnInit function once, followed by the OnTick function, which will cycle indefinitely for every tick. If the Expert Advisor is halted manually or due to an event, it will then initialize the function OnDeInit and terminate the algorithm.
The foundation of an Expert Advisor
- OnInit: Operates only once when the Expert Advisor begins.
- OnTick: Runs forever and executes its instructions whenever a new tick or price movement happens.
- OnDeinit: Runs only once before the Expert Advisor is completely stopped.
How are the indicators evaluated?
The Variables that define indicators parameters are initialized during the execution of the function OnInit() which runs once at the loading of the Expert Advisor. As value from indicators must be calculated for each price tick, the logic of the algorithm should be enclosed in the function OnTick(), which is the main event-handling function that iterates at every price movement. For instance (Fig.116), the Moving Average data point is assessed at every tick movement to determine whether the current price is above or below the Moving Average. In the Fig.116 case, the condition to buy is when the price is above the Moving Average and sell when the price is below. OnTick() will repeat the process indefinitely unless the Expert Advisor is terminated.
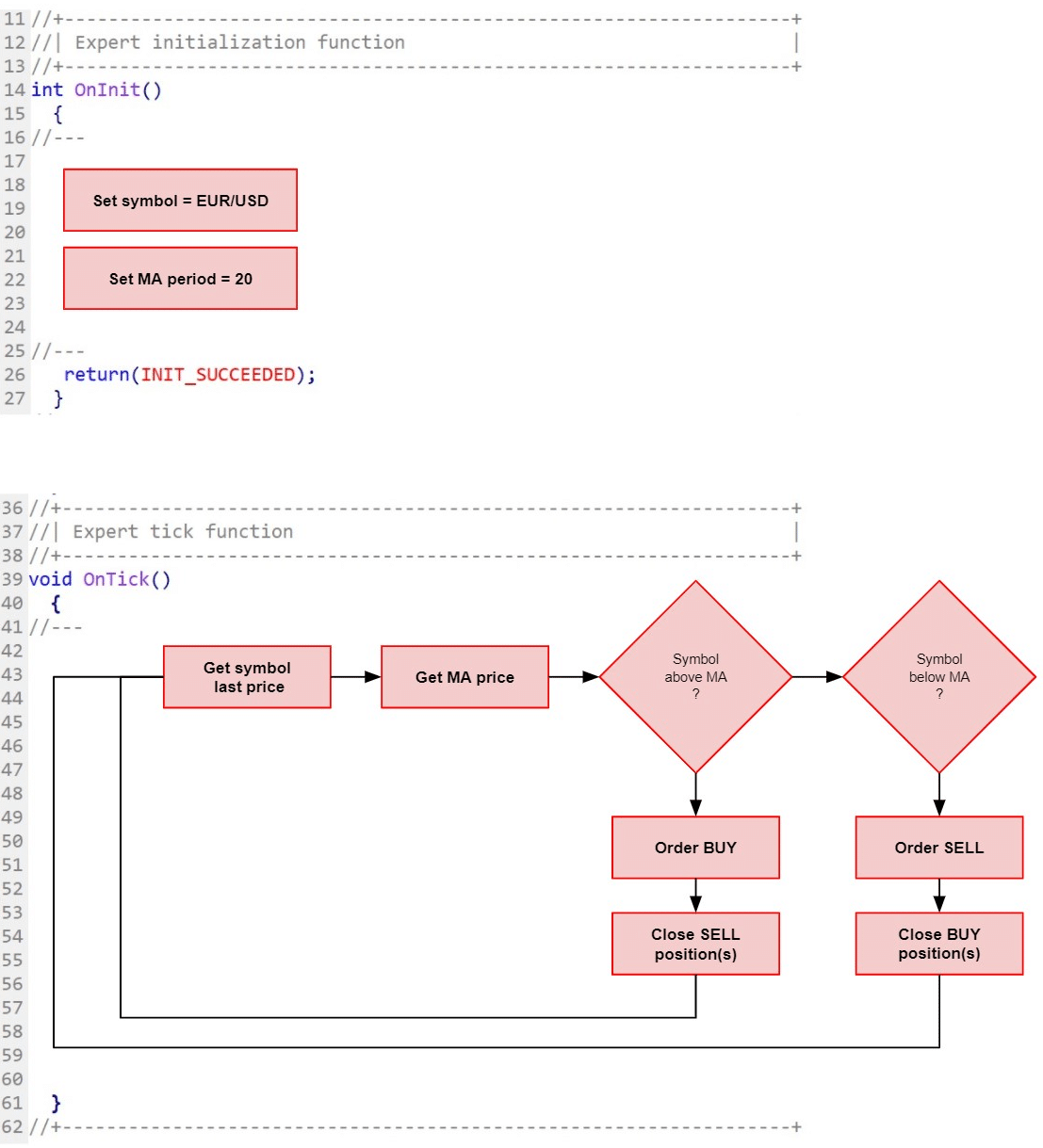
Case study: Create a strategy with the MQL5 wizard and going through the code structure
Let us take a real case scenario of a trading strategy with a combination of signals from the Moving Average 20-period and the Relative Strength Index 14-period (Fig.117). The objective of this demonstration is to show you how to create a basic trading algorithm that combines two technical indicators. We will not go into the details of the algorithm logic but will explain to you the structure of how an Expert Advisor operates. For that, we will take this strategy for our case study:
- Buy when the price is above the Moving Average (20-period), and the RSI (14-period) is below the 50 level.
- Sell when the price is below the Moving Average (20-period), and the RSI (14-period) is above the 50 level.
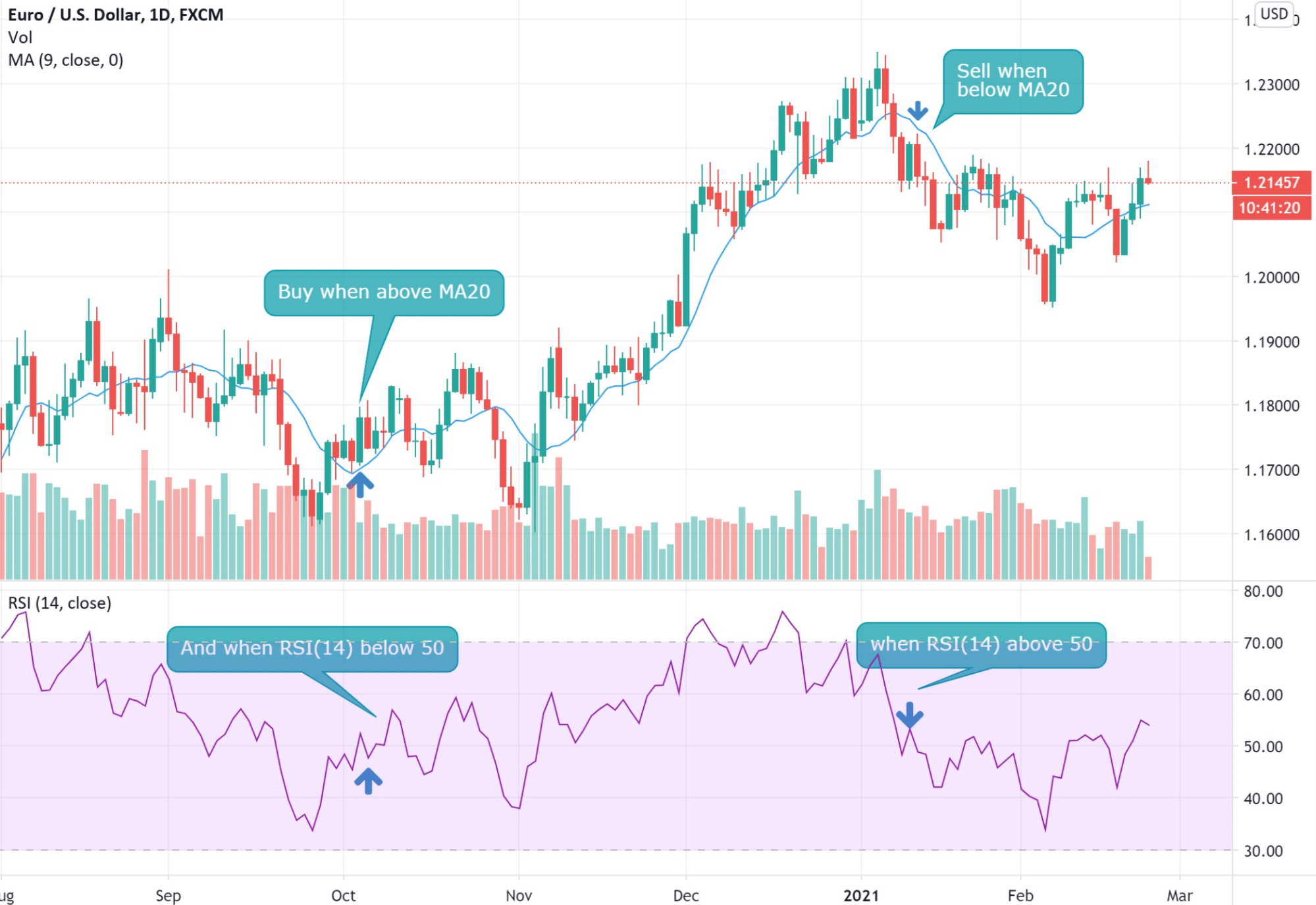
![]() |
From the MetaEditor, click on the menu “New” to create a new Expert Advisor. Choose the options Expert Advisor (generate). |
![]() |
Provide a name to your Expert Advisor and keep all the parameters as default, then press “Next.” |
![]() |
Click on the “Add” button to select the technical indicator “Moving Average.” Set the parameter “PeriodMA” to 20 to set the indicator to 20-period. Press “OK” to save your settings. |
![]() |
Repeat the same process as for the “Moving Average” indicator, but this time select “Relative Strength Index.” Set the parameter “PeriodRSI” to 14.
Press “OK” to save the settings. |
Once you have gone through all the steps of the MQL5 Wizard, you will see the generated code of the algorithm, we will then go through the most important lines with some explanations.
Let us go through the code.
Line 9 – 19: MQL5 wizard had attached and embedded into the code some standard libraries that are necessary to run the code. Libraries are a piece of code that is stored in other files. The principle of using libraries is to be able to reuse the code for many projects without having to rewrite multiple times.
Line 12: Expert.mqh is a core library and is used to manage the mechanism of the expert advisor.
Line 14 – 15: Signal libraries are libraries that manage signals derived from technical indicators. In our case, for MA and RSI.
Line 17: Trailing library is to manage trailing stops if you have any implemented.
Line 19: “Money” library is to manage all the aspects of money management such as contract volume and lot size.
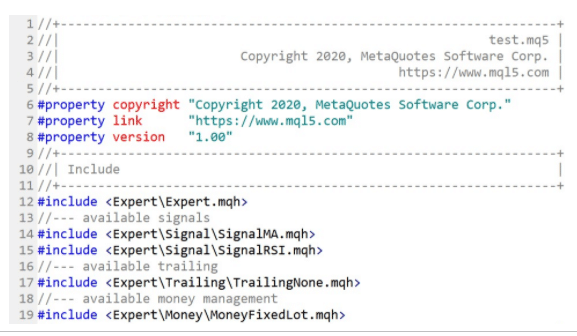
Where to find the Expert library?
Expand the list from the navigator panel. Under the folder “Include,” you will find the subfolder “Expert” which hosts all the libraries that relate to Expert Advisor. If you double click on the .mqh file, you will be able to see the code of the selected library.
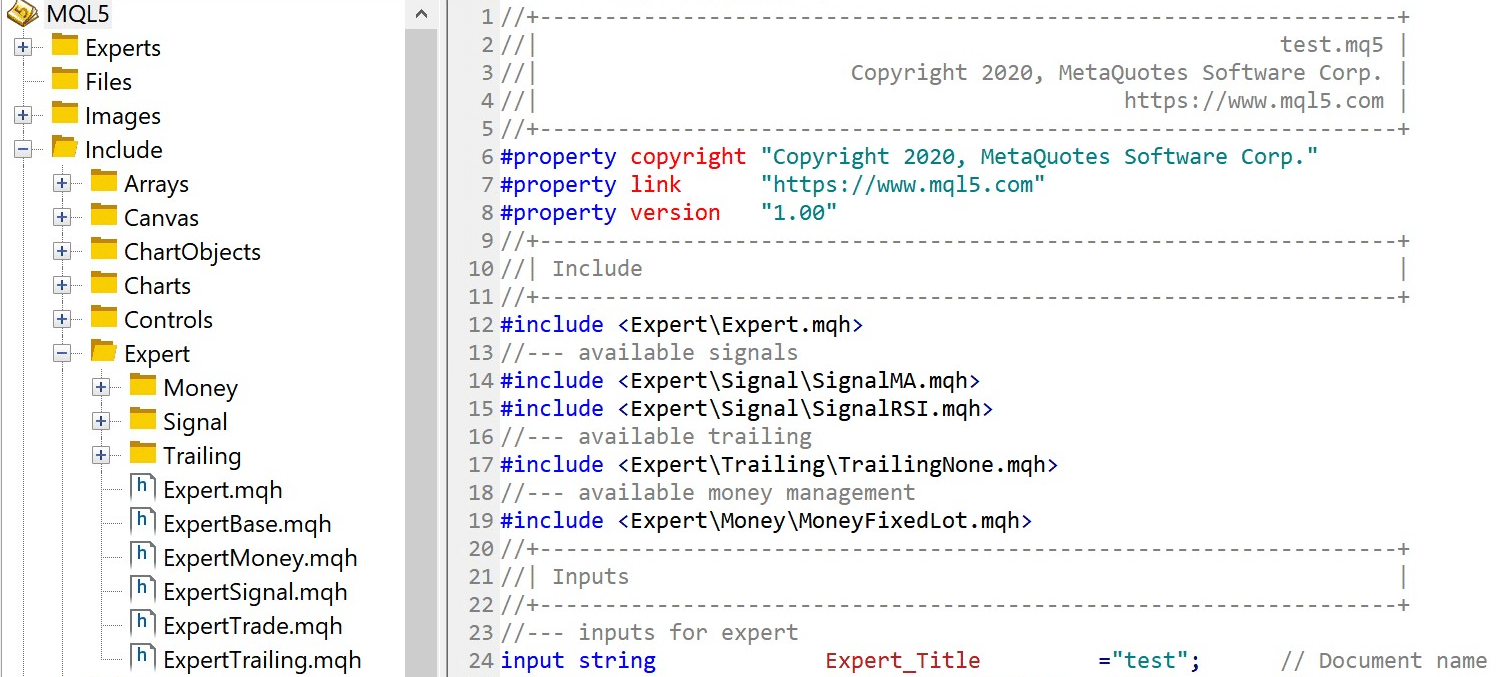
Declaration of global input variables
Line 24 – 44: All the configurable variables are declared in this section. Note that input variables can be modified in MetaTrader, Expert Advisor properties. Values that are assigned in the code are default values.
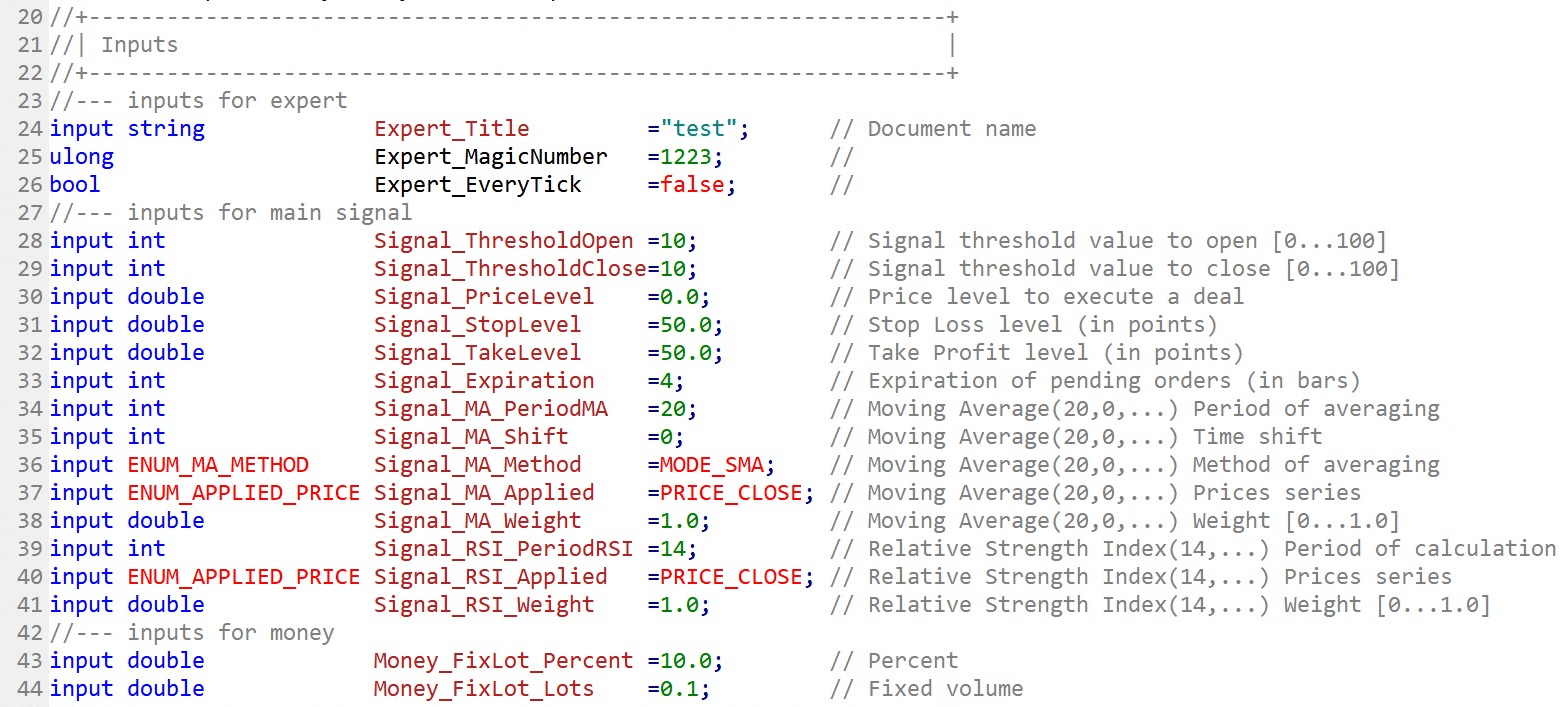
Declare the global expert object
Line 48: The expert object belongs to the library Expert in the file Expert.mqh. You may refer to line 12. The Expert object is the main object that will handle all the mechanisms of the algorithm.

Initialize the Expert Advisor
The OnInit() function runs only once when the Expert Advisor loads, therefore, the enclosed code will only run one time.
Line 54 – 61: The Init() function from the Expert object is called to initialize the expert object with parameters provided by the input variables, for instance, Expert_EveryTick and Expert_MagicNumber.
Line 62 – 70: Declare the object Signal of the library that handles the signal. “Signal” is basically the library that controls the outcome of a signal according to technical indicators. In our case MA and RSI.
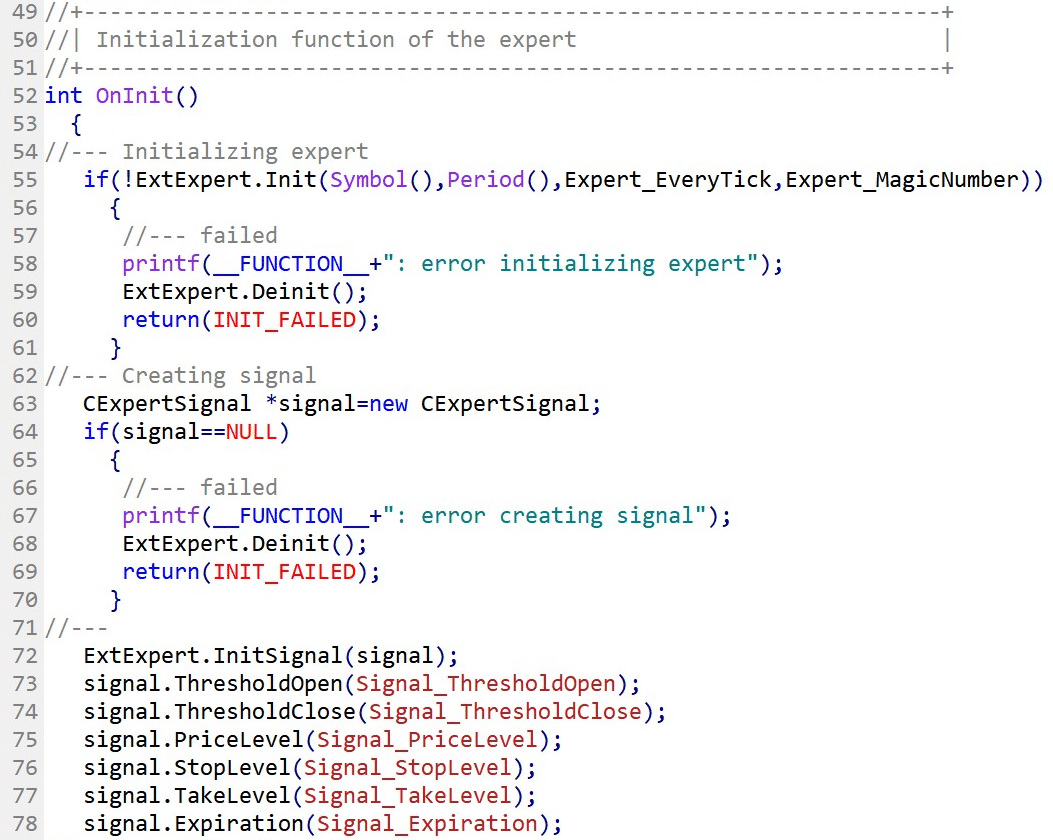
Initialize technical indicators parameters
Line 88 – 108: Call the AddFilter() from the object signal to define the parameters for the technical indicators, MA, and RSI provided by the input variables.
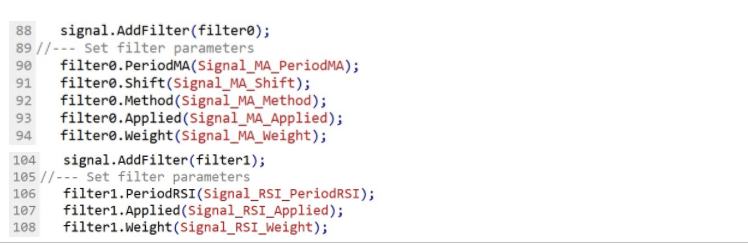
The iteration at every tick
The OnTick() function will execute the enclosed code at every generated tick price.
Line 177: The OnTick() function from the expert object is called to execute the main function of the Expert Advisor.
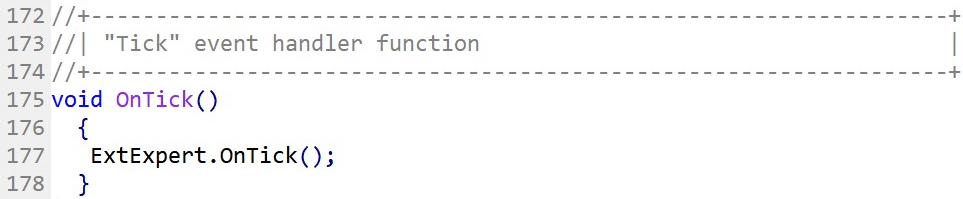
The main function that contains the logic of the algorithm
The onTick() function is calling the OnTick() of the object “expert” to perform the logic of the algorithm. To view the details of the code of the OnTick(), go to the navigator panel and scroll through the list of libraries to locate the Expert.mqh file. Double click on it to open the code file.
Note: It is important to not alter the library code. Libraries may be used in other projects and as we mentioned, libraries are reusable code. Therefore, if you modify the code, you may face some unexpected errors in other Expert Advisor projects due to dependencies.
Line 642: You can search through the code to locate the function CExpert::OnTick(void).
Line 651: The OnTick() function calls the function Processing() which will process the code that evaluates the logic of your expert advisor. Use the keyboard combination CTRL-F to search for the keyword “Processing(void)” in the Expert.mqh file (Fig.126).
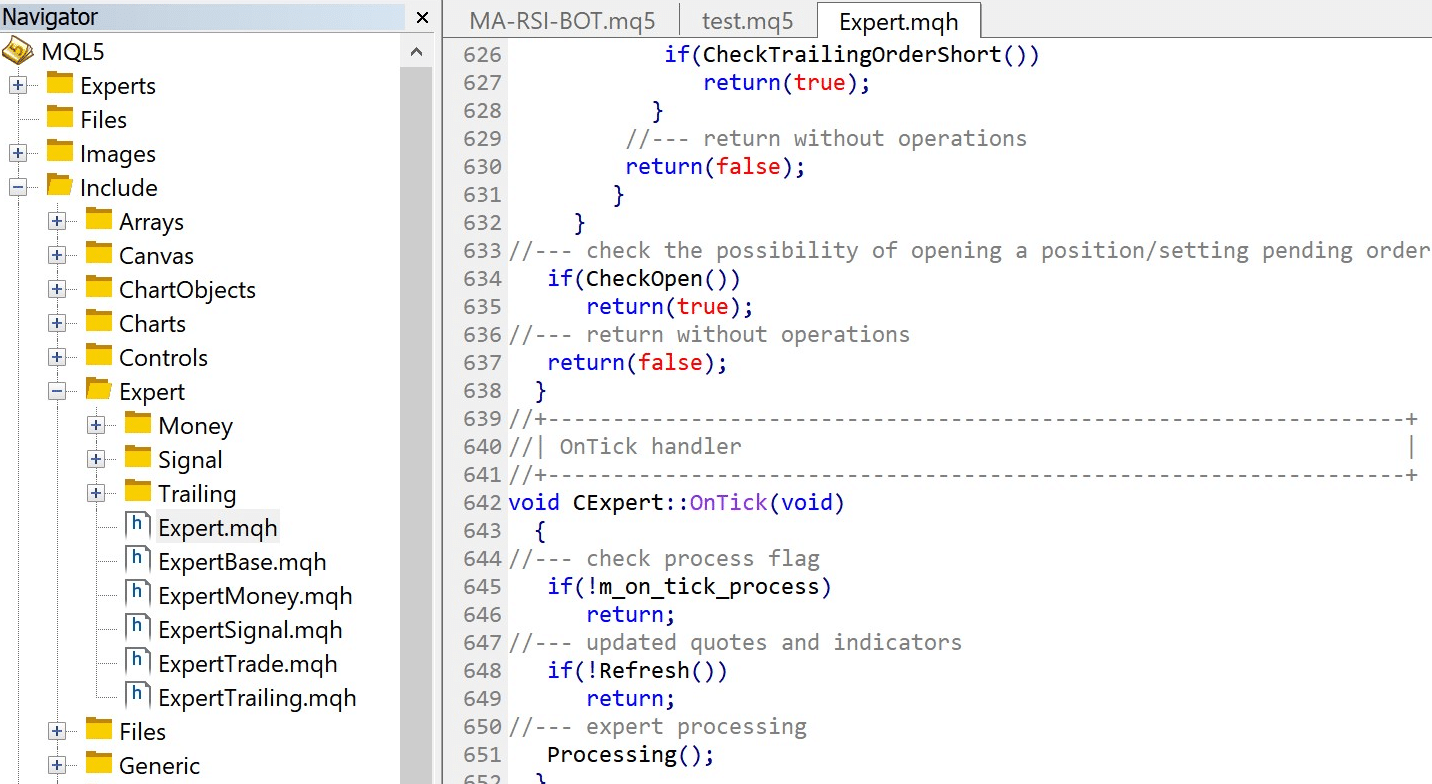
Expert object main function
Processing is the main algorithm that manages trade entry and exit. It handles the logic of the algorithm according to provided parameters and values initialized in the function OnInit().
Line 584: Signal direction is set according to technical indicators settings specified in OnInit().
Line 586 – 600: Verify if there are any open positions and manage its trailing stop.
Line 603 – 632: Manage pending orders.
Line 634 – 638: Open position if conditions are matching.
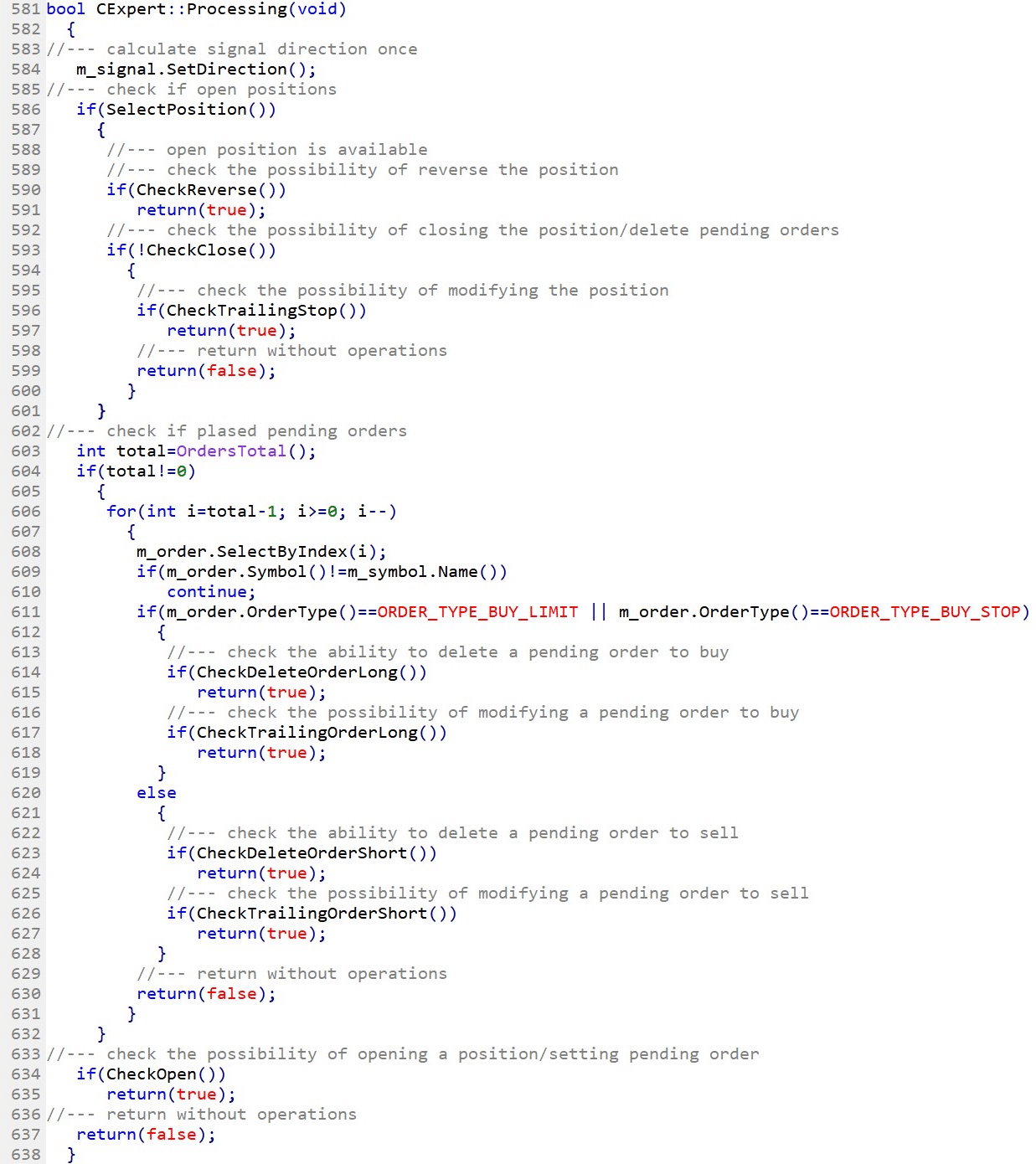
Coding tips:
If you are coding with MQL5, you can use the MQL5 Wizard to help you layout the basic structure of an indicator-based strategy algorithm. MQL4 does not have this MQL generator, however, the concept of OnInit() and OnTick() structure remains the same to build a trading algorithm.