The WHILE and DO WHILE statements are used to repeat a cycle of the block of instructions for an undefined number of times. The loop is interrupted once the termination criteria are met. There are 3 different ways to implement a loop in MQL4 and MQL5, WHILE, DO WHILE, and FOR statement. WHILE and DO WHILE share the same notion of a condition based on an undefined number of iterations contrasting with FOR statement, which is a loop with a specified start and end condition. Some practical examples of a loop that can be implemented with WHILE or DO WHILE are:
- Collect data in a file and append values in a dynamic array (Fig.90).
- Read a text file line by line for information.
- Find the price from the last MA20 and MA50 moving average crossover.
Note:
WHILE and DO WHILE statements share these same properties:
- The loop can repeat cycles of a block of instructions an unknown number of times.
- The loop can run on multiple termination conditions.
The only difference between WHILE and DO WHILE statements are:
- WHILE statement: the loop instructions are executed after the evaluation of the condition (Fig.87).
- DO WHILE statement: the loop instructions are executed before the evaluation of the condition for the loop to run (Fig.88).
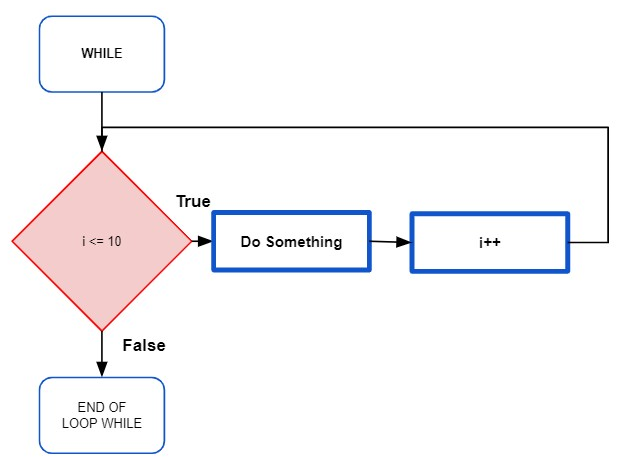
- Fig.87 Flowchart of a WHILE loop
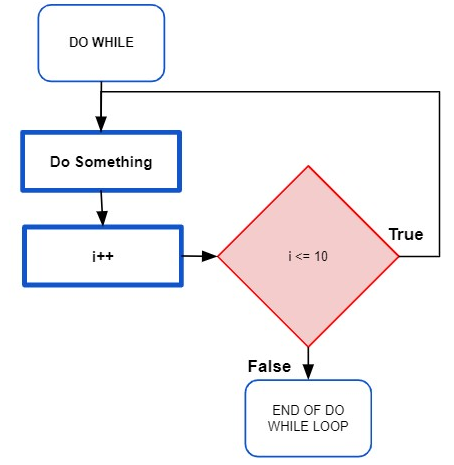
Unlike the FOR statement, WHILE and DO WHILE statement can use any expression to evaluate the criteria for the loop to run. Multiple conditions can be aggregated.
![]() |
![]() |
Fig.89 Code example of Fig.87 (left) and Fig.88 (right).
Fig.89 demonstrates the syntax difference between WHILE and DO WHILE statements. Fig.90 exhibits a real case scenario of the use of a WHILE loop to read a text file and append each line from the file to an array:
- Line 51-53: Declare variables that define the file to read.
- Line 56: Declare variable file_handle, which is the file location.
- Line 57: Declare the array that will store collected data from the file.
- Line 58: Declare the variable that will increment the index of the array, not for the loop.
- Line 60: Opening of the IF statement that checks that the file path is valid.
- Line 72-73: If the file path is not valid, the program will omit to file reading instructions and print an error in the MetaTrader log.
- Line 63: Repeat the loop cycle as long as the condition of FileIsEnding() is false.
- Line 65: Append to the data array cell the line content of the file.
- Line 66: Increment the array index.
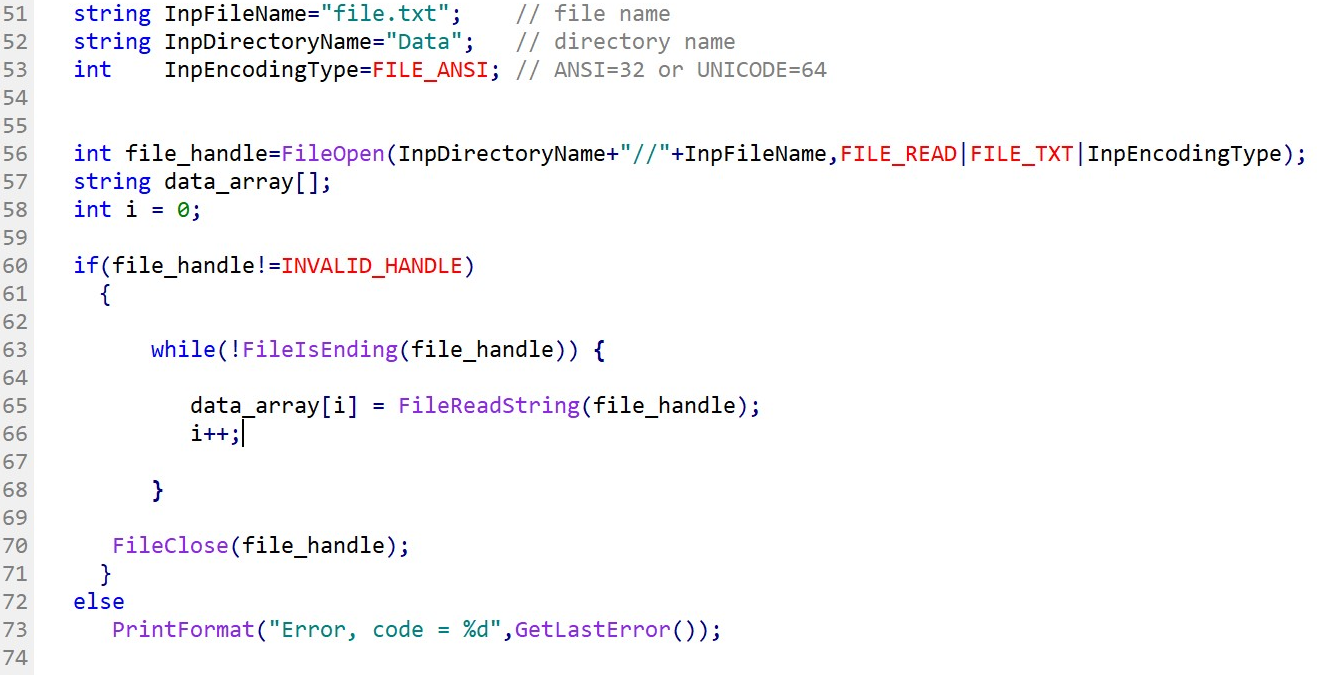
Anatomy of a WHILE statement
|
Anatomy of a DO WHILE statement
|
Fig.91 Anatomy of a WHILE and DO WHILE statement
WHILE statement has its condition defined first between parentheses. Conversely, DO WHILE statement has its condition described after the block of instructions. If the conditions are met or true, the loop will continue to run. On the contrary, the loop will be terminated. There are two reasons for a WHILE and DO WHILE loop to be terminated:
- The loop conditions are not met or false.
- The loop is interrupted by a control statement such as BREAK.
Note:
WHILE and DO WHILE statements allow the use of multiple conditions. The example below demonstrates the use of two variables iteration, variable a and variable c.
- Variable a is declared and initialized with the value of 5.
- Variable c is declared and initialized with the value of 1.
- The loop condition is it will run indefinitely while a is bigger or equal to 1 AND c is smaller or equal to 5.
- Note that variable a is decremented while variable c is incremented.
![]() |
![]() |
Case study: Which one can be an adequate use of the WHILE statement?
Case 1: Find the price of EUR/USD from the last time the RSI was at the level of 70.
Case 2: Find the latest lowest price points from the last 10 days.
Case 3: Calculate the average daily range from the last 50 days.
Case 4: Read the .csv file that contains economic data and import the content in a dynamic array.
Case 5: Get the largest divergence between EUR/USD and GBP/USD since the last 200 days.
ANSWER: Case 1 and case 4 are adequate candidates for the WHILE or DO WHILE statement. The reason is simply that the loop repeat cycle is not predefined or unknown. For instance, for case 4, we do not know how many lines are composing the .csv file and, therefore, the WHILE or DO WHILE statement is the most appropriate method to perform the loop.
Coding tips:
Make sure to define the loop condition that can be terminated to avoid an infinite loop. An infinite loop can crash your program and is a significant risk, particularly while developing a trading robot. Avoid the use of a global variable as an element for iteration.