The FOR statement is a loop that consists of a block of code that repeats a sequence of instructions until the termination condition is met. The FOR statement is used to iterate through values and to perform various operations such as calculations and data manipulations. Some practical examples of a loop that can be achieved with FOR loop are:
- Check what is the lowest price since the last 20-day period.
- Check if, since the last ten days, the MA20 and MA50 moving averages crossover occurred.
Note:
The FOR statement requires 3 expressions to operate (Fig.84):
The FOR statement is the only type of loop that requires three expressions to implement it. Instead, WHILE and DO WHILE statements only need the condition for the loop to run. |
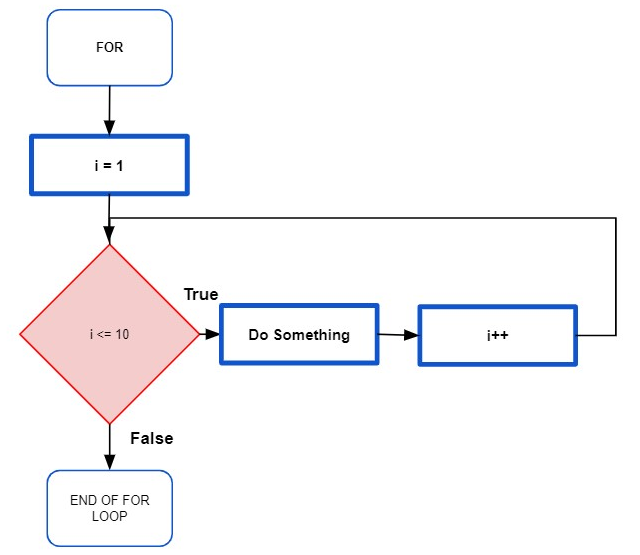
FOR statement evaluates the iteration variable prior to executing its instructions (Fig.84).
- As per illustration in Fig.84, the variable i is first initialized with the value of 1.
- The variable i is then assessed at every cycle with the expression: as long as i is smaller or equal to 10, keep repeating the enclosed instructions “Do Something.”
- The variable i is then incremented according to the iteration expression i++.
![]() |
![]() |
Fig.85 Code example of Fig.84. with to approach to declare the iteration variable i
Fig.85 demonstrates a typical FOR statement.
- int i; is the declaration of variable i, which will be used for the loop iteration.
- i = 1; is the initialization of the variable i to start at the value of 1.
- i <= 10; is the condition for the loop to run. If the answer is true, the loop will end.
- i++ is the incrementation of variable i.
- do_something(); is an example of a called function or instruction.
The variable i is incremented at every repeat cycle until i is NOT smaller and NOT equal to 10, as the condition for the loop to run is, repeat while i is smaller or equal to 10.
Anatomy of a FOR statement
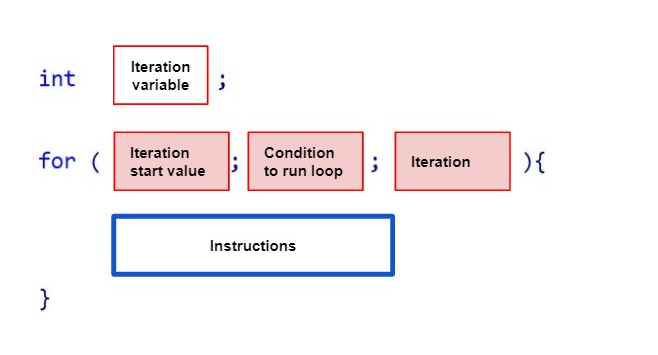
There are two methods to declare the iteration variable. It can be prior to the FOR statement or inside the first expression that defines the iteration start value (Fig.85). Three expressions must be provided in the following order separated by a semicolon:
- Attribution of the start value of the iteration variable.
- Condition for the loop to run.
- The increment or decrement of the iteration variable.
- If more than one instruction is provided in the repeat cycle, they must be enclosed inside curly brackets. Conversely, it is not necessary to employ curly brackets for one instruction.
Note:
- You can declare and use an iteration variable prior to the FOR statement.
- You can declare the iteration variable within the first expression of the FOR statement.
CASE STUDY: Which one can be an adequate use of FOR statement?
Case 1: Collect data in a file and add and save it in a dynamic array.
Case 2: Read a text file line by line for information.
Case 3: Check if there are any opened orders on EUR/USD and update variable EU_order_exist.
Case 4: Find the latest 5 highest price points from the last 50 days.
Case 5: Find the price from the last MA20 and MA50 moving average crossover.
ANSWER: Case 3 and case 4 can adequately use the FOR statement. The reason is the condition for the loop to run is precise with a known start and end, unlike case 1, case 2, and case 5, whose termination condition is unknown. In case 1 and case 2, the program is reading a file, and the number of lines in the file might be unknown. The termination condition is the end of the file.
Coding tips:
The most important aspect in implementing a loop is to make sure that the termination expression can be fulfilled. In a FOR statement, make sure that the loop condition is not an equal comparison but rather a limit. As per the examples below, statement 2 is the recommended form. (statement 1) FOR loop with termination condition set to variable i equal to N*
(statement 2) FOR loop with termination condition set to variable i bigger than N*
|