A SWITCH statement is similar to the IF and ELSE-IF statement. SWITCH is a selection control that depends on the condition provided by a single variable to control the algorithm branching. The main difference between SWITCH and ELSE-IF statement is that SWITCH statement condition is based on one variable while ELSE-IF can have conditions from multiple variables or multiple expressions.
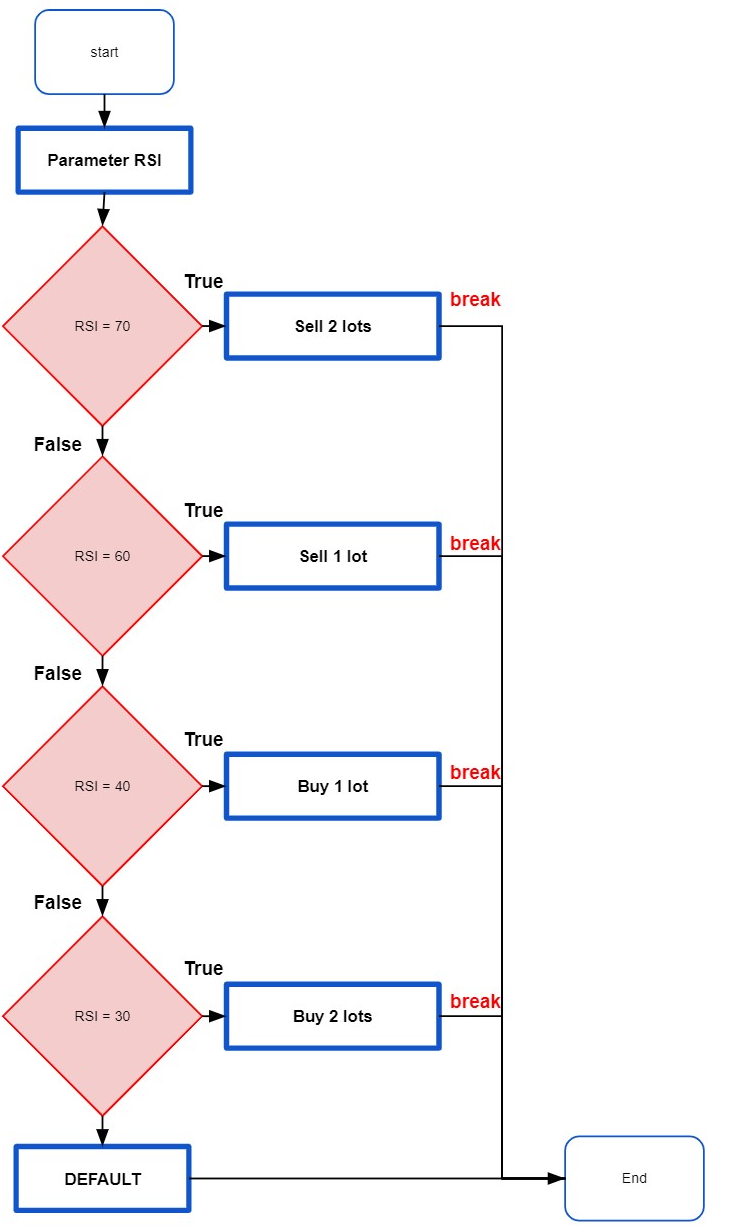
Fig.74 Demonstrates the control of algorithm branching according to the value of variable RSI.
- If the RSI variable is equal to 70, the program will execute a Sell of 2 lots, exit the SWITCH statement and continue its flow to the next program instruction.
- For each case variation of the RSI variable, the program will execute the instruction accordingly (For instance: if the RSI is equal to 60, the program will Sell 1 lot; if it is 40, the program will Buy 1 lot, etc.)
- If none of the cases is matching the condition, a default instruction will be attributed.
Fig.75 shows the program structure of Fig.74 in code. The program execution sequence evaluates the variable RSI, which is specified between parentheses. If none of the conditions are met for each case, a default instruction is implemented. In this case, the program will call the function Print(“No trade to execute!”).
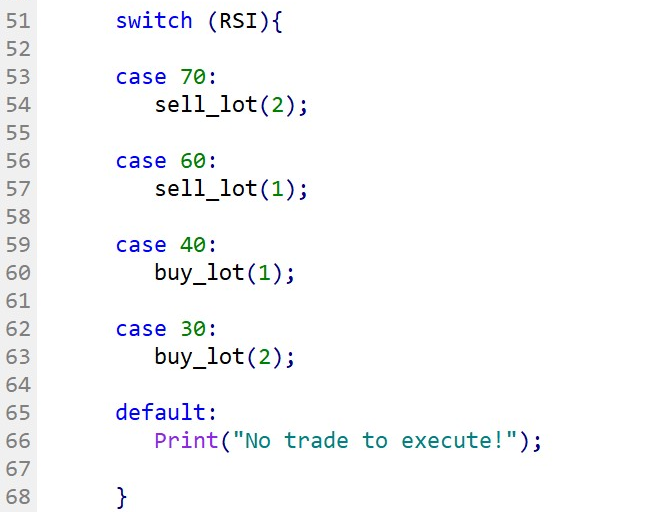
Coding tips:
The switch statement is useful to control complex conditional branching of operations. The switch transfers the control of the program to a statement within its body. Inheriting from the C language, SWITCH statement is mostly accompanied by a “break” instruction at the end of each “case.” It is recommended to implement it as illustrated in Fig.76. A break instruction is used to immediately terminate a program control. In this situation, after a “case” has been fulfilled, the program will exit the SWITCH statement block. As a best practice, the break instruction allows a faster program execution as once instructions have been executed, and the program will immediately exit and end the SWITCH statement. Conversely, without break instructions, the program will continue to assess each of the cases until the end of the SWITCH statement, which is inefficient. |
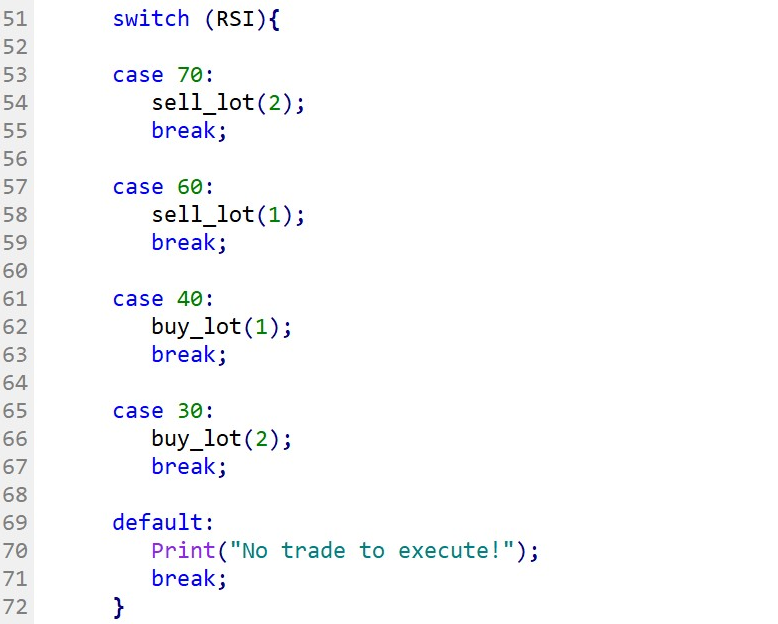
Anatomy of a SWITCH statement
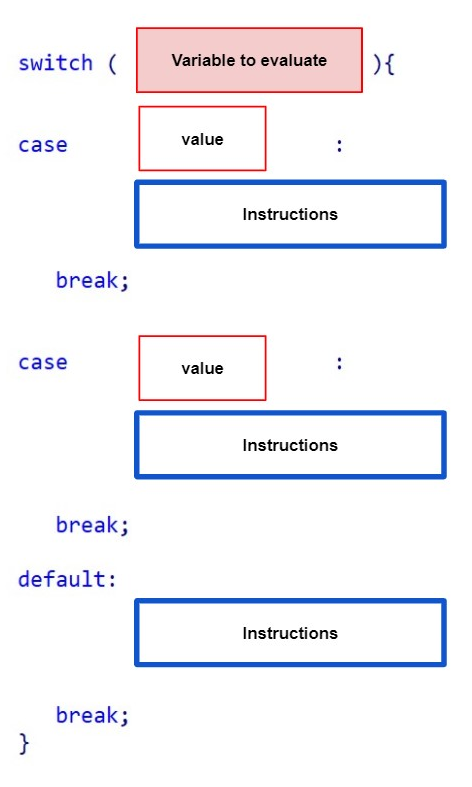
A SWITCH statement is very similar to an ELSE-IF statement; however, the SWITCH evaluates a single variable while ELSE-IF can assess conditions based on expressions and multiple variables. A SWITCH is a more graceful approach when assessing multiple values for a single variable.
- The first part of a SWITCH statement is the specification of the variable to evaluate. The variable must be declared and assigned with a value prior to the statement. The variable must be enclosed between parentheses.
- The SWITCH statement body should be enclosed between curly brackets and comprise at least one case and one default operator.
- The CASE must be followed by the value to assess according to the variable type and opened with a colon punctuation mark. Note that curly brackets should not be used to enclose CASE instructions even if there are more than one.
- The DEFAULT operator is used to provide a default instruction.
- BREAK instruction is recommended at the end of each CASE instruction.
Note:
|
Case study: Which solution is the most effective: ELSE-IF or SWITCH?
- The program needs to check whether the current price is above the Moving Average or the RSI indicator is below the 30 Level to execute a BUY trade.
ANSWER: An ELSE-IF statement will be more appropriate as more than one variable must be assessed to decide which instruction to execute.
- The program needs to check the distance between the Moving Average and the current price. If the current price is less than ten pips above the Moving Average, then instruct the program to execute a BUY trade. If it is above, the program should call for a SELL trade.
ANSWER: An IF statement will be appropriate as the program will have to evaluate an expression. Even though the expression is related to a single variable, the SWITCH cannot be used in this case as SWITCH statement can only assess precise values.
- The programme needs to check the time. When it is 9 am, the programme should execute a BUY order. At 1 pm, the programme should instruct another BUY order. When the day ends at 6 pm, the programme should close all the trades.
ANSWER: A SWITCH statement will be appropriate as the program will have to evaluate a single variable to decide which instruction to carry out.
Coding tips:
The SWITCH statement is useful to simplify your code. Instead of having a code with a long sequence of ELSE-IF statements, SWITCH allows the evaluation of a specified variable and executes instructions accordingly. |