A loop is a basic concept in any programming language. It is simply a question repeatedly asked until an answer is provided. Once an answer is satisfied, the program will quit the loop and continue its execution to the next instruction. In programming concepts, the asked question is called an iteration. For instance, the use of loop applied to algorithmic trading can be:
- Read historical data and determine which of the prices from X previous days have been the lowest until today.
- Read historical Moving Average prices of DMA 20 and DMA 50 indicators from X previous days and determine if a Moving Average crossover happened during that period.
- Browsing opened trade positions and verification if a particular trade reached profitability to set stop loss orders to break even for specific trades that meet a particular criterion.
- Constantly check opened trade positions and trail stop loss for trades that reached a certain threshold.
- Calculate the total profit and loss from the last X number of closed trades.
Note:
There are many types of loops, MQL4 and MQL5 language has three of them:
If the argument or the criteria to end the loop is never met, the loop will be endless unless the program is forced to stop or a loop control statement such as BREAK is initiated inside the loop. Loops can be nested to appear inside another loop regardless of its type, FOR, WHILE, or DO WHILE. |
It is important to remember these peculiarities that differentiate the FOR, WHILE, and DO WHILE statements. The FOR and WHILE loop operator are both checking the loop termination expression at the beginning before each cycle. On the contrary, the DO WHILE statements check the condition at the end of each loop iteration. It means that instructions in each of the cycles are executed before any termination. By default, loops are designed to repeat a set of instructions sequentially until the termination argument is met or the reason for termination is true. However, loop control statements can be used to alter the execution of a cycle by forcing the interruption of the loop or by forcing the loop to go to the next iteration.
FOR loop
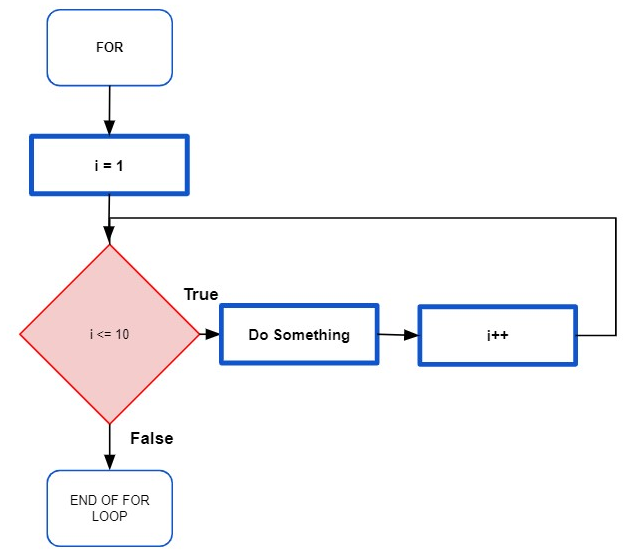
Unlike WHILE and DO WHILE loop, the FOR loop requires three expressions to define it:
- The initialization of an integer is used as a variable for iteration.
- The condition for the loop to run.
- The expression that defines the iteration, increase, or decrease of the iteration variable.
WHILE loop
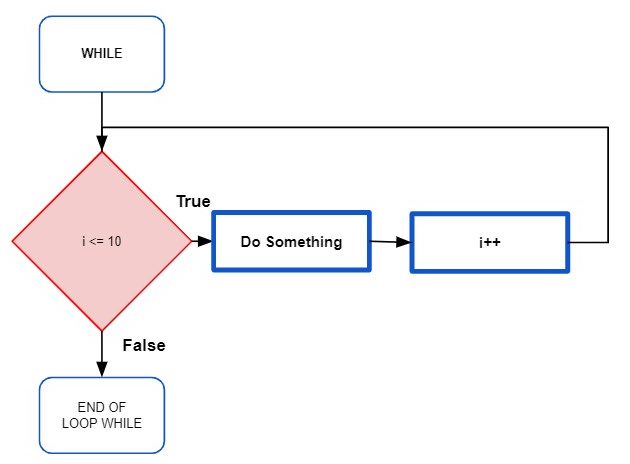
WHILE loop just requires the condition for the loop to run. Instructions are executed after the evaluation of the condition, which is like FOR loop in terms of functionality. The WHILE loop can use any expressions to evaluate the criteria for the loop to run, unlike the FOR statement that requires an integer as a variable for the iteration.
DO WHILE loop
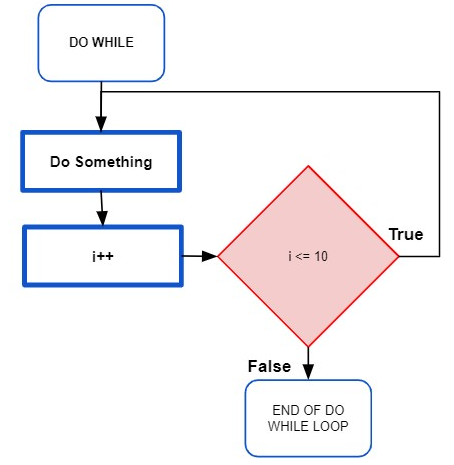
DO WHILE loop is different to both FOR and WHILE statement as instructions are executed before evaluation of the condition for the loop to run.
Loop control statements
|
BREAK control statement
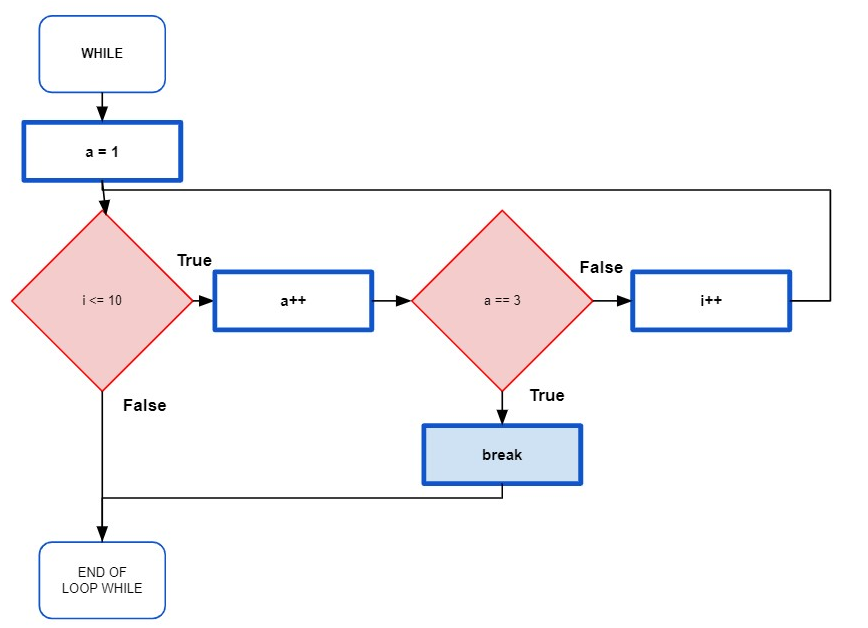
CONTINUE control statement
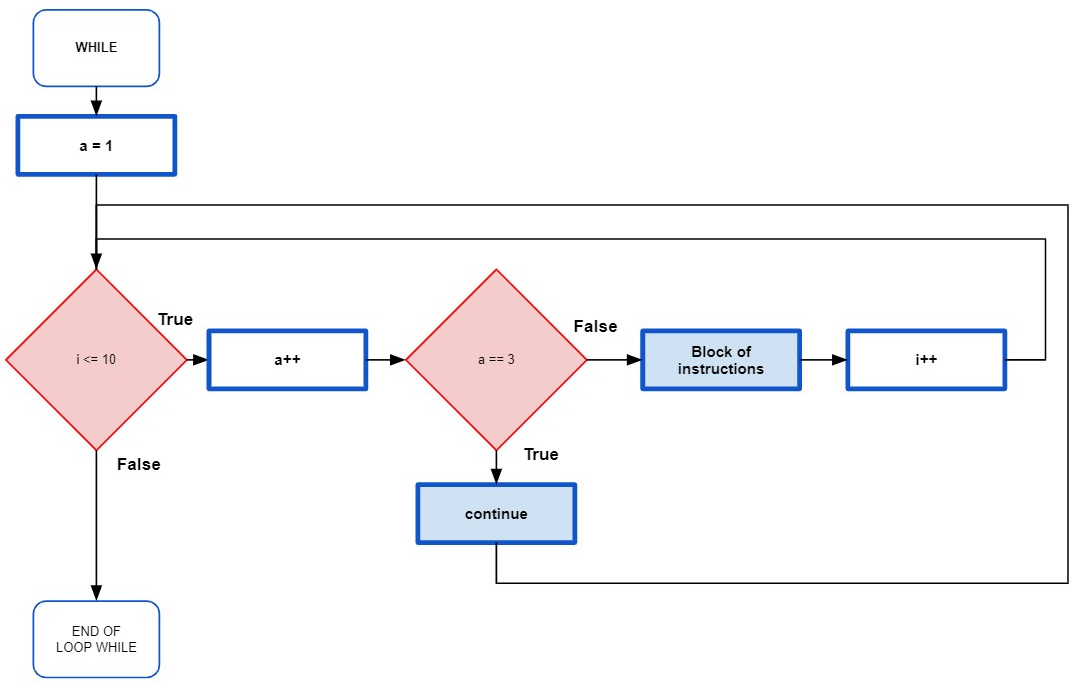
Case Study: Which Loop statement is an infinite dead loop?
One of these loops in Fig.83 is a never-ending loop; can you recognize it?
Loop A
|
Loop B
|
Fig.83 Two examples of the loop statement
Answer: Loop A because the loop termination expression cannot be fulfilled, and the variable i can never be equal to five. Therefore, the loop will repeat indefinitely as the condition is:
- Repeat if variable i is not equal to five.
- The variable i value is changed to six during the cycle where i is equal to four.
Coding tips:
Always make sure to implement a loop termination expression that can be fulfilled. Otherwise, you might encounter a dead loop. Here are two examples of correctly written FOR loop which are very similar and will in normal circumstances produce the same result, but one of them is a reliable approach and recommended. (statement 1) FOR loop with termination condition set to variable i equal to N*
(Statement 2) FOR loop with termination condition set to variable i bigger than N*
Statement 2 is the recommended form to write a reliable loop. The reason is that whatever happens during the loop execution, the termination expression will be fulfilled regardless of the value of the variable i if i is greater than N. On the other hand, the termination expression provided in statement 1 might not be fulfilled as for the loop to end, the variable i must be exactly equal to N. For instance if i is incremented while it is as well updated during the execution, the variable i may never be equal to N which can cause an infinite loop (Fig.83). |